Introduction
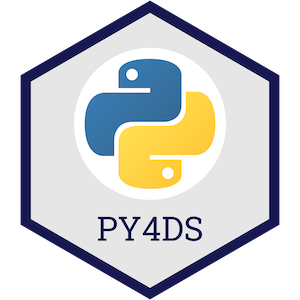
This topic material is based on the Python Programming for Data Science book and adapted for our purposes in the course.
Exercise¶
What is 5 to the power of 5?
# Your answer here.
Exercise¶
What is the remainder from dividing 73 by 6?
# Your answer here.
Exercise¶
How many times does the whole number 3 go into 123? What is the remainder of dividing 123 by 3?
# Your answer here.
Exercise¶
Split the following string into a list by splitting on the space character:
s = "MDS is going virtual!"
# Your answer here.
Exercise¶
Given the following variables:
thing = "light"
speed = 299792458 # m/s
Use f-strings to print:
The speed of light is 2.997925e+08 m/s.
# Your answer here.
Exercise¶
Given this nested list, use indexing to grab the word "MDS":
l = [10, [3, 4], [5, [100, 200, ["MDS"]], 23, 11], 1, 7]
# Your answer here.
Exercise¶
Given this nest dictionary grab the word "MDS":
d = {
"outer": [
1,
2,
3,
{"inner": ["this", "is", "inception", {"inner_inner": [1, 2, 3, "MDS"]}]},
]
}
# Your answer here.
Exercise¶
Why does the following cell return an error?
t = (1, 2, 3, 4, 5)
t[-1] = 6
Exercise¶
Use string methods to extract the website domain from an email, e.g., from the string "davi.moreira@fakemail.com"
, you should extract "fakemail"
.
email = "davi.moreira@fakemail.com"
# Your answer here.
Exercise¶
Given the variable language
which contains a string, use if/elif/else
to write a program that:
- return "I love snakes!" if
language
is"python"
(any kind of capitalization) - return "Are you a pirate?" if
language
is"R"
(any kind of capitalization) - else return "What is
language
?" iflanguage
is anything else.
language = "python"
# Your answer here.
!jupyter nbconvert _01-py-basics-practice.ipynb --to html --template classic --output 01-py-basics-practice.html